アンドロイドのあれこれ
[PR]
×
[PR]上記の広告は3ヶ月以上新規記事投稿のないブログに表示されています。新しい記事を書く事で広告が消えます。
NFC Handson、タグを読み取る
今日は、NFCタグの基本の情報を読み取る簡単なサンプルを紹介します。
読み取る情報はタグの、アクション、ID、TechListです。
NFCのパーミッションを追加するのを忘れずに
AndroidManifast.xml
読み取る情報はタグの、アクション、ID、TechListです。
NFCのパーミッションを追加するのを忘れずに
AndroidManifast.xml
<uses-permission android:name="android.permission.NFC" />
MainActivity.java
端末にNFCを有効にし、アプリ立ち上げてNFCタグにかざしてみてましょう。
アプリ起動していてタグにかざした瞬間、
onPause -> onNewIntent -> resolveIntent -> onResume
の順番にメソッドが呼ばれます。
インテントフィルターを追加
AndroidManifest.xml
どのタグタイプに対応するかをnfc_tech_filter.xmlに定義します
res/xml/nfc_tech_filter.xml
インテントを受けたらタグの情報を表示させるためにonCreateにインテントを受け取るように書きます。
MainActivity.java
タグかざしたらこのようなイメージになります。
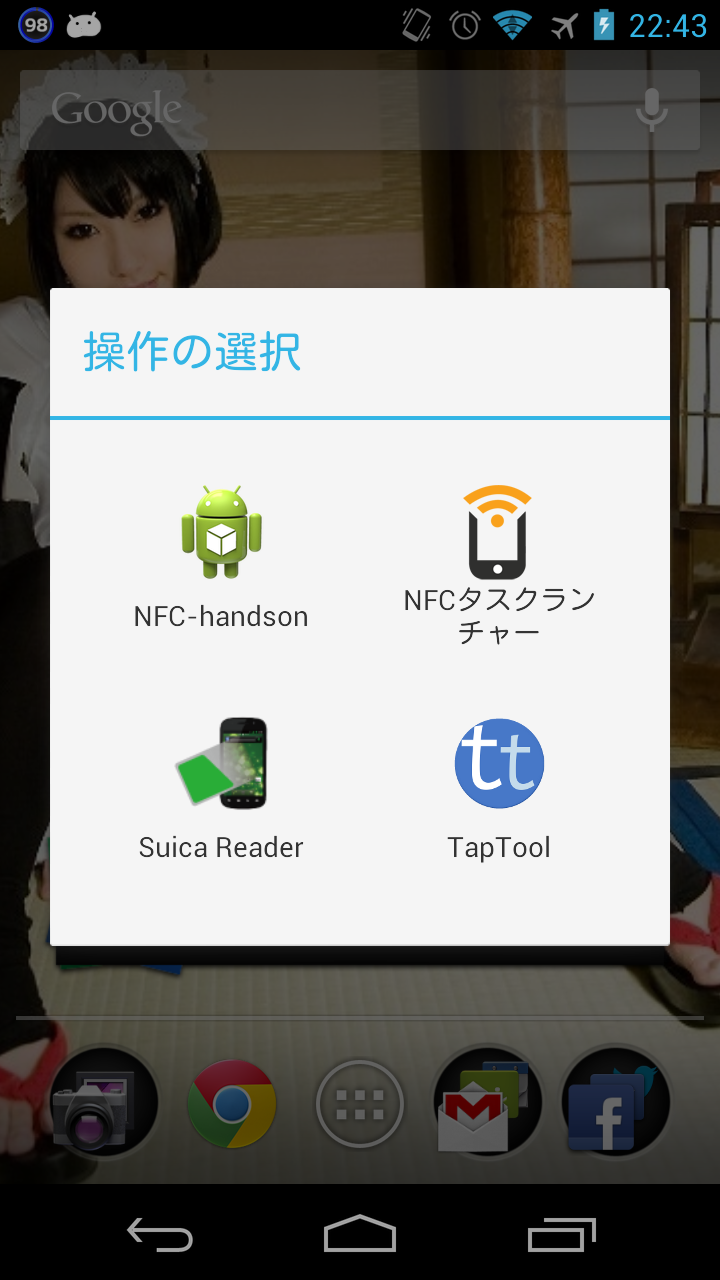
以上のすべてのソースコードはGithubに公開しています。
public class MainActivity extends Activity {
private NfcAdapter mAdapter;
private PendingIntent mPendingIntent;
private IntentFilter[] mFilters;
private String[][] mTechLists;
TextView nfcActionView;
TextView nfcIdView;
TextView nfcTagView;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
//タグの情報を表示するためのビュー
nfcActionView = (TextView)findViewById(R.id.nfc_action_view);
nfcIdView = (TextView)findViewById(R.id.nfc_id_textview);
nfcTagView = (TextView)findViewById(R.id.nfc_tag_view);
//NFCアダブター取得
mAdapter = NfcAdapter.getDefaultAdapter(this);
mPendingIntent = PendingIntent.getActivity(this, 0,
new Intent(this, getClass()).addFlags(Intent.FLAG_ACTIVITY_SINGLE_TOP), 0);
IntentFilter tag = new IntentFilter(NfcAdapter.ACTION_TAG_DISCOVERED);
mFilters = new IntentFilter[] { tag, };
mTechLists = new String[][] { new String[] { NfcF.class.getName() } };
}
public void resolveIntent(Intent intent){
String action = intent.getAction();
if (isNFCAction(action)) {
//アクションを表示
nfcActionView.setText(action);
byte[] rowId = intent.getByteArrayExtra(NfcAdapter.EXTRA_ID);
String text = bytesToText(rowId);
//タグのIDを表示
nfcIdView.setText(text);
Tag tag = (Tag)intent.getParcelableExtra(NfcAdapter.EXTRA_TAG);
String techStr = "";
for (String tech : tag.getTechList()) {
techStr = techStr + tech + "\n";
}
if (techStr.equals("")) {
techStr = "no techList.";
}
//TechListを表示
nfcTagView.setText(techStr);
}
}
//NFCのアクションかを判断する
private boolean isNFCAction(String action){
if (action.equals(NfcAdapter.ACTION_TECH_DISCOVERED)
|| action.equals(NfcAdapter.ACTION_TAG_DISCOVERED)
|| action.equals(NfcAdapter.ACTION_NDEF_DISCOVERED) ) {
return true;
}
return false;
}
//バイト値の変換
private String bytesToText(byte[] bytes) {
StringBuilder buffer = new StringBuilder();
for (byte b : bytes) {
String hex = String.format("%02X", b);
buffer.append(hex).append(" ");
}
String text = buffer.toString().trim();
return text;
}
@Override
public void onResume() {
super.onResume();
if (mAdapter != null) {
//フォアグラウンドディスパッチを有効に
mAdapter.enableForegroundDispatch(this, mPendingIntent, mFilters,
mTechLists);
}
}
@Override
public void onPause() {
super.onPause();
if (mAdapter != null) {
//フォアグラウンドディスパッチを無効に
mAdapter.disableForegroundDispatch(this);
}
}
@Override
public void onNewIntent(Intent intent) {
setIntent(intent);
resolveIntent(intent);
}
}
端末にNFCを有効にし、アプリ立ち上げてNFCタグにかざしてみてましょう。
アプリ起動していてタグにかざした瞬間、
onPause -> onNewIntent -> resolveIntent -> onResume
の順番にメソッドが呼ばれます。
・事前にアプリを起動せずにNFCタグかざしてインテントを受け取る
次はインテントフィルターでタグにかざしたときにアプリ指定して(対応しているアプリが複数ある場合)選択できるようにします。つまりアプリを起動しなくてもNFCに反応させることです。インテントフィルターを追加
AndroidManifest.xml
...
<activity
android:name=".MainActivity"
>
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<intent-filter>
<action android:name="android.nfc.action.TECH_DISCOVERED" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
<intent-filter>
<action android:name="android.nfc.action.TAG_DISCOVERED" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
<meta-data android:name="android.nfc.action.TECH_DISCOVERED"
android:resource="@xml/nfc_tech_filter" />
</activity>
...
どのタグタイプに対応するかをnfc_tech_filter.xmlに定義します
res/xml/nfc_tech_filter.xml
<?xml version="1.0" encoding="utf-8"?>
<resources xmlns:xliff="urn:oasis:names:tc:xliff:document:1.2">
<tech-list>
<tech>android.nfc.tech.NfcF</tech>
</tech-list>
<tech-list>
<tech>android.nfc.tech.NfcA</tech>
</tech-list>
<tech-list>
<tech>android.nfc.tech.NfcA</tech>
<tech>android.nfc.tech.MifareClassic</tech>
<tech>android.nfc.tech.Ndef</tech>
</tech-list>
<tech-list>
<tech>android.nfc.tech.NfcF</tech>
<tech>android.nfc.tech.Ndef</tech>
</tech-list>
</resources>
インテントを受けたらタグの情報を表示させるためにonCreateにインテントを受け取るように書きます。
MainActivity.java
...
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
nfcActionView = (TextView)findViewById(R.id.nfc_action_view);
nfcIdView = (TextView)findViewById(R.id.nfc_id_textview);
nfcTagView = (TextView)findViewById(R.id.nfc_tag_view);
mAdapter = NfcAdapter.getDefaultAdapter(this);
mPendingIntent = PendingIntent.getActivity(this, 0,
new Intent(this, getClass()).addFlags(Intent.FLAG_ACTIVITY_SINGLE_TOP), 0);
IntentFilter tag = new IntentFilter(NfcAdapter.ACTION_TAG_DISCOVERED);
mFilters = new IntentFilter[] { tag, };
mTechLists = new String[][] { new String[] { NfcF.class.getName() } };
//インテントを受け取ったら
if (getIntent().getAction() != null) {
resolveIntent(getIntent());
}
}
タグかざしたらこのようなイメージになります。
以上のすべてのソースコードはGithubに公開しています。
COMMENT